Get premium membership and access questions with answers, video lessons as well as revision papers.
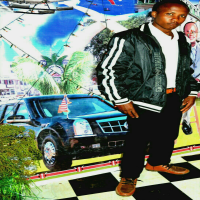
//Dynamically allocated stack.
#include
#include
using namespace std;
// Declare a stack class for characters
class stack {
char *stck; //holds the stack
int tos; // index of top of stack
int size; // size of the stack
public:
stack (int s) ; //constructor
~stack(); destructor
void push ( char ch ); //push character on stack
char pop (); // pop character from stack
};
// Initialize the stack
stack::stack (int s)
{
cout << "constructing a stack\n";
tos = 0;
stck = (char *) malloc (s);
if(!stck) {
cout << "Allocating error\n";
exit (1);
}
size = s;
}
stack::~stack()
{
free(stck);
}
// Push a character.
void stack::push (char ch)
{
if(tos==size) {
cout << "stack is full\n;
return;
}
stck[tos] = ch;
tos++;
}
if (tos==0) {
cout << "Stack is empty\n";
return 0; // return null on empty stack
}
tos--;
return stck [tos];
}
int main()
{
// Create two stacks that are automatically initialized.
stack s1 (10), s2(10);
int i;
s1.push ('a');
s2.push ('x');
s1.push ('b');
s2.push ('y');
s1.push ('c');
s2.push ('z');
for (i=0; i<3; i++) cout << "pop s1: "<< s1.pop() << "\n";
for(i=0; i<3; i++) cout << "pop s2: " <return 0;
}
Githiari answered the question on May 5, 2018 at 17:48
- Create a C++ class called stopwatch that emulates a stopwatch that keeps track of elapse time. Use a constructor to initially set the elapse time...(Solved)
Create a C++ class called stopwatch that emulates a stopwatch that keeps track of elapse time. Use a constructor to initially set the elapse time to 0.Provide two member function called start() and stop() that turns on and off the timer, respectively. Include a member function called show() that displays the elapsed time.Also, have the destructor function automatically display elapsed when stopwatch object is destroyed.
Date posted: May 5, 2018. Answers (1)
- Create an overload rotate() function using C++ new style that left-rotates the bits in its argument and returns the result.Overload it so it accepts ints...(Solved)
Create an overload rotate() function using C++ new style that left-rotates the bits in its argument and returns the result.Overload it so it accepts ints and longs.(A rotate is similar to a shift except that the bit shifted off one end is shifted onto the other end)
Date posted: May 5, 2018. Answers (1)
- Create a class in C++ language that holds name and address information.Store all the information in character strings that are private members of the class.Include...(Solved)
Create a class in C++ language that holds name and address information.Store all the information in character strings that are private members of the class.Include a public function that stores the name and address.Also include a public function that displays the name and address. (Call these functions store() and display() )
Date posted: May 5, 2018. Answers (1)
- Write a program that uses C++ style I/O to prompt the user for a string and then display its length.(Solved)
Write a program that uses C++ style I/O to prompt the user for a string and then display its length.
Date posted: May 5, 2018. Answers (1)
- Given the following new-style C++ program, show how to change it into its old-style form.#include using namespace std;into f(into a);into main(){cout << f(10);return 0;}int f(int...(Solved)
Given the following new-style C++ program, show how to change it into its old-style form.
#include
using namespace std;
into f(into a);
into main()
{
cout << f(10);
return 0;
}
int f(int a)
{
return a * 3.1416;
}
Date posted: May 5, 2018. Answers (1)
- Create a function in C++ language called rev_str() that reverses a string.Overload rev_str() so it can be called with either one character array or two.When...(Solved)
Create a function in C++ language called rev_str() that reverses a string.Overload rev_str() so it can be called with either one character array or two.When it is called with one string, have that one string contain the reversal.When it is called with two strings, return the reversed string in second argument.For example
char s1[80], s2[80];
strcpy (s1, "hello");
rev_str(s1, s2; //reversed string goes in s2, s1 untouched
rev_str(s1); //reversed string is returned in s1
Date posted: May 5, 2018. Answers (1)
- Outline four main steps in computer forensics(Solved)
Outline four main steps in computer forensics.
Date posted: May 4, 2018. Answers (1)
- Properties of a firewall (Solved)
Properties of a firewall
Date posted: May 4, 2018. Answers (1)
- Define controversial content(Solved)
Define controversial content.
Date posted: April 28, 2018. Answers (1)
- State the methods of verification giving examples(Solved)
State the methods of verification giving examples
Date posted: April 28, 2018. Answers (1)
- What is verification? (Solved)
What is verification?
Date posted: April 28, 2018. Answers (1)
- Why is authentication important?
(Solved)
Why is authentication important?
Date posted: April 28, 2018. Answers (1)
- State the examples of biometric devices (Solved)
State the examples of biometric devices
Date posted: April 28, 2018. Answers (1)
- What is authentication?
(Solved)
What is authentication?
Date posted: April 28, 2018. Answers (1)
- Why do we need privacy?
(Solved)
Why do we need privacy?
Date posted: April 28, 2018. Answers (1)
- How does computer technology threaten the privacy of our data? (Solved)
How does computer technology threaten the privacy of our data?
Date posted: April 28, 2018. Answers (1)
- Define privacy(Solved)
Define privacy
Date posted: April 28, 2018. Answers (1)
-
State the types of Intellectual Property Protection. (Solved)
State the types of Intellectual Property Protection.
Date posted: April 28, 2018. Answers (1)
- Define law(Solved)
Define law
Date posted: April 26, 2018. Answers (1)
- Define Ethics (Solved)
Define Ethics
Date posted: April 26, 2018. Answers (1)